Contents of source.php:
Several points were gathered from the encoder function:
- $enc_tab, an array, comprised of 91 items.
- $var1 contained the (shifted) ASCII value of the input character being processed.
- $var2 contained the number of bits to be processed.
- $var3 contained the working value of $var1.
- If $var1 was between 89 and 8191, and $var2 was more than 13, its value would be shifted right by 13 places, otherwise its value would be shifted right by 14 places. 2 characters would be picked from $enc_tab based on the quotient and remainder from the division operation.
Flag captured! 200 points in the bag!! Yay!!!
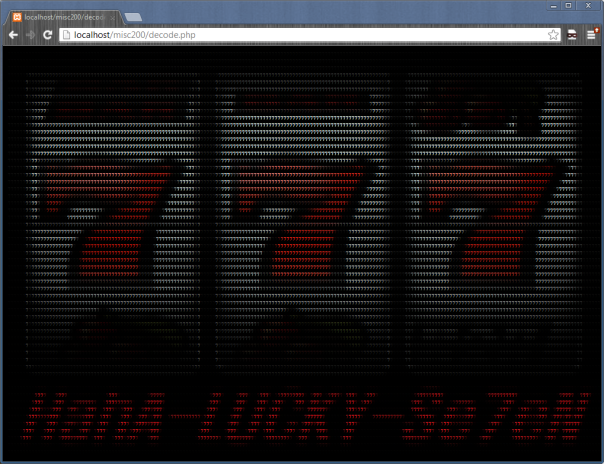
Cheers,
Braeburn Ladny
No comments:
Post a Comment